How to parse JSON in Android?

How to parse JSON in Android?
Always having troubles when you want to JSON in Android. How I download the .json file from the internet? How can i parse the JSON into a java model? How can we build this on a stable way? With this post we hope to answer all those questions!
As JSON API we are using content from jsonplaceholder.typicode.com, an free fake Online REST API.
Before we start we first need to prepare the Android project with the libraries we are using: RxJava, RxAndroid, OkHttp and GSON. Any information about RxJava, RxAndroid or OkHttp is not needed to follow this how to, for any background information several other posts are already available.
For this example we are downloading the JSON data from the internet. Step #1 for any internet activities within Android is to set the permission "android.permission.INTERNET" in the AndroidManifest.xml.
How to download JSON content from the web?
Android is quite strict in network operations, they are not allowed on Main thread. To prevent that the complete app is blocked on network activity, Android throws an exception "android.os.NetworkOnMainThreadException".
With below code sample we call from the onCreate directly parseExampleOfJsonObject with an url. Inside parseExampleOfJsonObject Rxjava is used in a stream, also RxJava is used to prevent the NetworkOnMainThreadException by running all HTTP traffic on another thread. OkHttp is used inside downloadJson to build and execute the HTTP request and directly return the parsed the body. The parsed body is actually a string with all the json content.
When you run below code a log line will be printed showing the json content.
How to parse the JSON content?
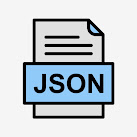
In order to parse JSON content we use GSON, an free lib from Google which can map Java objects directly on their JSON representation.
Gson is a Java library that can be used to convert Java Objects into their JSON representation. It can also be used to convert a JSON string to an equivalent Java object. Gson can work with arbitrary Java objects including pre-existing objects that you do not have source-code of.
First we are defining a Java model based on the JSON structure, within this example it is Todo.java. This class contains the same fields as defined within the JSON.
The convert action is execute by GSON, when called with the following parameters:
Todo todo = gson.fromJson(json, Todo.class);.
A Todo object is created which can be used to read all object fields. In this example we print the Todo object into logcat.
Does this also work for a JSON list?
Yes, by providing TypeToken.
A TypeToken is an generic type T, which means it can be any type even with generics like a list for example.
Type listType = new TypeToken<List<Todoo>o>() {}.getType();
By providing the listType in gson.fromJson(json, listType) it returns a List of Todo objects (ArrayList<Todo>). See below for the complete code sample. In this example we print the first two objects from the list towards logcat.
Links
- RxJava: Reactive Extensions library
- RxAndroid bindings for Android library
- OkHttp: Modern HTTP client library
- Gson library
With this guideline everybody can download json webpages and deserialize them with GSON!
Thank you for reading this article, If you like this article, help others find this article by sharing it.
Reacties
Een reactie posten